Mastering RAG Implementation: Covering all the Basics
Retrieval-augmented generation improves big language models by using external sources of information to provide accurate and context-rich responses. This blog post discusses RAG's architecture, implementation steps, practical applications, model kinds, and advantages over fine-tuning.
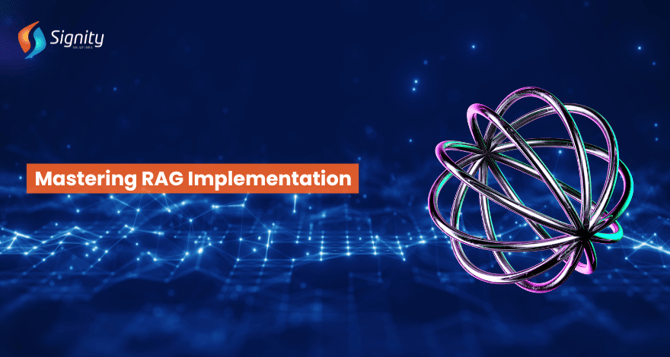
With the introduction of Large language models, Artificial intelligence has progressed significantly. Chatbots and other automated content generators are powered by these models, creating new opportunities in natural language processing. Despite their excellence, these models frequently have issues with relevance and precision, which can be problematic when details count.
Here comes the retrieval-augmented generation services (RAG) for rescue. RAG can include data from external knowledge sources, such as databases and articles, into the text generation process, expanding upon models such as GPT. This indicates that the AI is forming its answers based on accurate, trustworthy facts rather than merely speculating.


- Retrieval-augmented generation optimizes LLMs by incorporating data from external knowledge sources.
- Implementing RAG includes data chunking, vector embeddings, and contextually relevant response generation for faster and more relevant results.
- Different RAG models exist, such as Basic, Multimodal, interactive, domain-specific, and adaptive.
- Unlike fine-tuning, RAG optimizes the LLMs with real-time knowledge, making it feasible for context-relevant responses.
In this post, we'll examine the implementation of RAG, its practical implementations, and its potential to enhance our interactions with AI systems by making them more dependable and helpful.
A Quick Introduction to RAG
A large language model's output is optimized by a retrieval-augmented generation (RAG) technique, which consults a reliable knowledge base outside its training data sources before producing a response.
LLMs are trained on enormous amounts of data and employ billions of parameters to create unique output for complex tasks like answering questions, translating languages, and finishing sentences.
Without requiring the model to be retrained, RAG expands the already potent capabilities of LLMs to specific domains or an organization's knowledge base. It is an economical method of enhancing LLM output to ensure accuracy and relevant context and is helpful in various settings.
What is the Process of Implementing Augmented Generation (RAG)?
RAG aims to provide language models with the data they require. Accurate facts are extracted from a well-maintained knowledge library before using that context to return the response instead of asking LLM directly (as in general-purpose models).
The retriever uses vector database embeddings, or numerical representations, to obtain the desired document when the user submits a query. The result is returned to the user after the necessary data is in the vector databases.
This refreshes the model without retraining it, which is very expensive and significantly lowers the chances of hallucinations.
Essential Components Of the RAG Architecture Model
The following are the essential components of the RAG Architecture model.
1. User Interaction
The client asks the system questions to start the procedure. The workflow as a whole begins with this user query.
2. Semantic Search in Vector Database
A semantic search mechanism communicating with a vector database processes the user query. Contextual data in this database is represented as vectors, which makes information retrieval adequate and relevant.
3. Contextual Information and Prompt Creation
The contextual data obtained is then used to generate a prompt. This prompt is used to provide information to the large language model.
4. Large Language Model
Equipped with the prompt, the LLM produces a response. The capabilities of the LLM guarantee that the reaction is informative, contextually relevant, and cohesive.
5. Post-Processing
A post-processing framework improves the output following the LLM's answer generation. This stage guarantees the finished answer is perfect and prepared for client distribution.
Workflow Of RAG Implementation
Let's go through the workflow of the RAG implementation process.
Step 1: Data Gathering
The first step is to collect all the information required for the application. A product database, a list of frequently asked questions, and user manuals can all be included in a company's customer care chatbot.
Step 2: Data Chunking
Dividing your data into smaller, easy-to-manage parts is known as data chunking. For example, if your user manual is 1000 pages long, you may divide it into sections that address distinct client questions.
In this manner, every data chunk is targeted at a particular topic. Since it doesn't include irrelevant information from complete documents, information received from relevant chunks of the source dataset is more likely to provide contextually relevant responses to the user query.
Efficiency is also increased because the system can extract the most relevant data rather than process whole documents.
Step 3: Embedding Documents
The original data must be transformed into a vector representation after being divided into smaller components. To do this, text data must be converted into embeddings, numerical representations in vector space that capture the semantic meaning of language.
To put it simply, document embeddings enable the system to comprehend user queries and, rather than relying just on word-to-word comparison, utilize the meaning of the text to match them with relevant chunks in the source dataset. This approach guarantees that the answers are suitable and in line with the user's request.
Step 4: Query Processing
The end user asks the system a question. This feeds the query into the framework, starting the process.
- The user submits a query (e.g., "What is Retrieval-Augmented Generation?").
- The user query is converted into a vector embedding using an embedding model (e.g., OpenAI embeddings, BERT, Sentence Transformers).
Step 5: Document Retrieval
- The retriever searches for the most relevant information from an external knowledge base (e.g., FAISS, Pinecone, Weaviate, or Elasticsearch).
- The retrieval method can be:
- Lexical search (BM25, keyword-based)
- Semantic search (vector similarity search using embeddings)
- Hybrid search (combining both lexical and semantic search for better accuracy).
Step 6: Augmenting the Query
The retrieved documents are concatenated with the user’s original query to provide context to the language model.
Step 7: Response Generation
After processing the prompt, the LLM produces a response. The LLM generates high-quality reactions since it has been extensively trained on large datasets. The model applies natural language understanding (NLU) and contextual reasoning to form an accurate and relevant answer to the user input or query.
Step 8: Returning the Response
Post-processing is applied to the resulting response to guarantee relevance, coherence, and clarity. This stage could entail improving the response's quality, fixing mistakes, and polishing the language.
Check out this detailed guide to Understand RAG:Architecture, Techniques, Use Cases, & Development
Practical Implementation Of Retrieval Augmented Generation
Step 1: Install Dependencies
|
Step 2: Load Embeddings & Store in FAISS
|
Step 3: Retrieve Relevant Documents
query = "What is RAG?" retrieved_docs = vector_db.similarity_search(query, k=2) for doc in retrieved_docs: print(doc.page_content) |
Step 4: Augment and Generate Response
|
Practical Uses of Retrieval-Augmented Generation
7 Practical Uses for RAG Models Retrieval-augmented generation models have proven adaptable in various fields. Several practical uses for RAG models include:
1. Complex Question-Answering Mechanisms
Question-answering systems that can retrieve relevant information and produce precise answers can be powered by RAG models, improving information accessibility for both people and enterprises. RAG models, for instance, can be used by a healthcare organization. By extracting data from medical literature and producing accurate answers, they may create a system that responds to medical inquiries.
2. Chatbots and Conversational Agents
Conversational agents are improved by RAG models, which enable them to retrieve relevant information from external knowledge sources. This feature guarantees that virtual assistants, chatbots for customer support, and other conversational interfaces provide precise and educational answers during interactions. In the end, it improves how well these AI systems support users.
3. Analysis and Research in Law
RAG models facilitate legal research procedures by locating relevant legal data and helping attorneys create documents, evaluate cases, and develop arguments more quickly and accurately.
4. Content Recommendation Systems
Enhance user experience and content engagement by using retrieval capabilities, analyzing user preferences, and creating personalized recommendations to power sophisticated content recommendation systems across digital platforms.
5. Content Summarization And Creation
In addition to streamlining content creation by obtaining relevant data from various sources and making it easier to produce excellent articles, reports, and summaries of specialized data, RAG models are also excellent at producing cohesive text in response to predetermined prompts.
These models are helpful for text summarization because they can extract relevant information from sources and provide concise summaries. A news agency, for instance, can use RAG models. They may be used to automatically generate news items or summarize long reports, demonstrating how versatile they are in supporting academics and content makers.
6. Resources and Tools for Education
Personalized learning is revolutionized by RAG models integrated into educational tools. By skillfully retrieving and creating customized explanations, questions, and study materials, they improve the educational process by meeting the needs of each individual.
7. Information Acquisition
RAG models improve search results' accuracy and relevancy, which benefits information retrieval systems. With the help of RAG models, which combine generating capabilities with retrieval-based strategies, search engines may also retrieve documents or web pages based on user queries. Additionally, they can produce educational tidbits that accurately convey the information.
Related Read: 10 Real-World Examples of Retrieval Augmented Generation
5 Different Models Of Retrieval Augmented Generation(RAG)
Examining various RAG model types, distinctive features, and typical use cases. Understanding RAG models can vary greatly depending on the requirements and goals. Each model employs traditional methods or a different method of retrieving and integrating data depending on the specific task or industry you're looking at.
Model 1: Basic RAG
In its most basic form, the RAG model responds to client inquiries by retrieving data from a particular knowledge base. This is most frequently employed when adding current information to the LLM's responses, such as through customer support chatbots or simple research tools, is the primary priority.
Model 2: Domain-Specific RAG
LLMs can be trained to extract Industry-specific facts from custom databases using RAG, guaranteeing that the responses accurately represent the domain knowledge required for highly specialized industries like law, financial services, and healthcare.
Model 3: Multimodal RAG
A multimodal RAG model can include data in formats other than text, including visuals and audio. Media analysis and instructional tools are two fields that greatly benefit from a wider variety of data inputs.
Model 4: Interactive RAG
Interactive RAG models can remember the context and history of the interaction to ensure each response is relevant and contextual for more complicated use cases that call for a conversational back-and-forth with the user, such as chatbot responses or virtual assistants.
Model 5: Adaptive RAG
Adaptive RAG models enable the LLM to learn from every contact and gradually enhance its information retrieval and operations in highly dynamic contexts where it must quickly adjust to new material or updated user preferences. This is useful in situations like personalized content delivery systems or predictive analytics.
How Does RAG Differ from Fine-Tuning LLM?
RAG and fine-tuning are critical strategies for expanding an LLM's knowledge and capabilities beyond its original training data. Although they both seek to enrich a pre-trained language model with domain-specific knowledge, they vary in their implementations.
Fine Tuning
A language model that has already been trained is fine-tuned using more training data for more domain-specific information about the task. This process takes place after NLP tasks. This method entails modifying the model's internal parameters in a particular body of knowledge, like use cases for a given industry.
Fine-tuning works well when the primary objective is to get the language model to carry out a particular activity, like analyzing client sentiment on distinct social media channels. Success in these situations does not require integration with such a specialized database as a knowledge repository or a great deal of external knowledge.
Fine-tuning, however, depends on static data, which is not optimal for use cases that rely on data sources that require updating or are subject to frequent change. Retraining an LLM such as ChatGPT on fresh data with every update is impossible from a financial, temporal, and resource perspective.
Retrieval Augmented Generation
RAG enhances the responses produced by the pre-trained language model by integrating non-parametric data from secondary knowledge sources using a retrieval mechanism with the model's pre-existing parametric memory.
RAG is a preferable option for knowledge-intensive tasks where real-time stock data or consumer behavior data are examples of external sources from which current information can be retrieved.
These assignments entail generating a summary by synthesizing intricate, detailed material or responding to open-ended, confusing inquiries. Additionally, fine-tuning carries a security risk. You risk disclosing private or sensitive information as you feed the model data. RAG grounding aids in removing this risk.
The Best Practices to Implement RAG
Effective RAG implementation necessitates a prudent strategy to guarantee its longevity. Consider the following steps in some areas:
1. Model Training
To adjust the RAG model to changing information and language usage, periodically retrain it using fresh datasets. Establish metrics to track accuracy, relevance, and biases in the responses, tools, and processes for continuously observing the model's output.
2. Better User Experience
Optimize the user experience by creating user interfaces that are simple to use and straightforward so that all users can access the system. Ensure the company you've partnered with for AI development services provides intelligible, concise, and clear responses.
3. Maintaining Data Quality
Schedule frequent data refreshes and keep the RAG model's data sources up to date to keep the information updated. Utilize a variety of reliable databases, journals, and other relevant platforms to source data to minimize bias.
Make Your AI Answer Smartly With RAG
Work with our RAG experts to build a system that provides appropriate answers.
4. Scalability Planning
Build the RAG system architecture to support scaling up from the beginning, considering variables like growing data volume and user load. To manage demanding data processing, provide enough resources for computing infrastructure, such as internal servers or cloud-based options.
5. Testing and Implementing Feedback
Establish procedures for collecting and incorporating all user input and feedback into continuous system enhancements. Before deployment, thoroughly test the RAG system in various real-world settings to guarantee its dependability.
6. Professional collaboration
To ensure the system is based on state-of-the-art knowledge, collaborate closely with data scientists and AI researchers. Promote cooperation between technical and non-technical teams using a comprehensive strategy combining domain-specific knowledge with AI experience.
7. Ethical Consideration
Implement strict procedures for data security, privacy, and compliance with data protection regulations. Maintaining awareness of and adherence to AI ethics and rules may entail making system adjustments and performing routine audits.
Accelerate Your Business with Signity’s RAG Enhanced AI
RAG stands out as a perfect solution as companies continue to be overwhelmed by data and need quicker, more precise solutions. At Signity Solutions, we utilize RAG's potential to create AI systems that are highly scalable, context-aware, and customized to meet your particular business requirements.
Our RAG development services guarantee that you're supported by the best of both worlds: deep retrieval and powerful generation, whether your goal is to improve customer service, optimize internal processes, or create intelligent applications.
Explore how our RAG Development Services can help you drive more innovative outcomes, faster decisions, and honest business value.
Frequently Asked Questions
Have a question in mind? We are here to answer. If you don’t see your question here, drop us a line at our contact page.
What is RAG Implementation?
An LLM's output is optimized by a retrieval-augmented generation technique, which consults a reliable external knowledge base outside its training data sources before producing a response.
What is Data Chunking?
Data chunking, especially in AI, big data, and cloud computing applications, divides large datasets or text into smaller, easier-to-manage pieces or chunks to increase processing speed and enhance model performance, memory utilization, and scalability.
What are Vector Databases?
What is the difference between Langchain and RAG Implementation?