Real-Time Translation in a Global Marketplace with OpenAI
Businesses today operate globally, necessitating effective communication across languages. OpenAI offers advanced language models enabling real-time translation. This article guides through leveraging OpenAI's tools, like GPT-3, for implementing translation in Python apps, fostering global market presence.
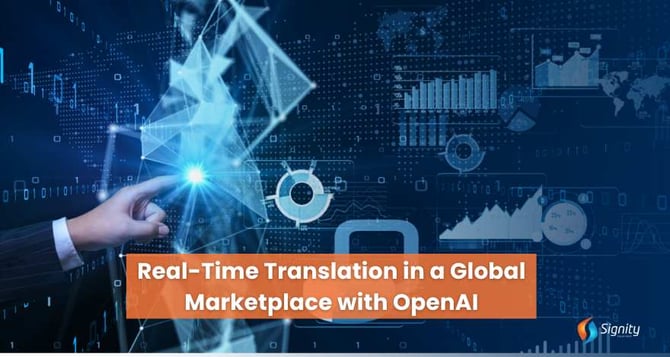
In today's interconnected world, businesses operate on a global scale, reaching customers and partners from different linguistic backgrounds. Effective communication is key to success in this global marketplace, and real-time translation plays a crucial role in breaking down language barriers.
OpenAI, a leader in artificial intelligence, offers powerful language models and APIs that can be leveraged to implement real-time translation solutions in your applications. In this article, we'll explore how to use OpenAI's tools and provide code snippets to enable real-time translation in a global marketplace.
Introduction to OpenAI's Language Models
OpenAI has developed state-of-the-art language models like GPT-3 and GPT-4. These models can perform a wide range of natural language understanding and generation tasks, including translation. With OpenAI's API, developers can easily integrate these models into their applications to enable real-time translation.
Getting Started
Before diving into the code, you'll need to set up an OpenAI account and obtain an API key. You can sign up for access to the OpenAI API on the OpenAI website.
Implementing Real-Time Translation with OpenAI
We'll build a simple real-time translation application using Python and OpenAI's GPT-3 model. In this example, we'll translate English text to French, but you can adapt the code for other languages as well.
Python Setup
First, make sure you have Python installed on your system. You'll also need to install the `openai` Python package using pip:
|
Real-Time Translation
|
In this code snippet:
- We import the `openai` module and set your API key.
- The `translate_text` function takes the input text, source language code (e.g., "en" for English), and target language code (e.g., "fr" for French) as arguments.
- We use OpenAI's GPT-3 model to generate a translation by providing a prompt that instructs the model to translate the input text.
- The translation is extracted from the response and returned.
Customization and Integration
You can customize this code to fit your specific application needs. For instance, you can build a user-friendly interface, support multiple languages, or add error handling. Additionally, you can explore other OpenAI models, like GPT-4, to further enhance translation quality.
Elevate Your Business with Custom AI Solutions
Our AI development services offer a tailored approach to meet your specific business needs. Let's discuss your project today!
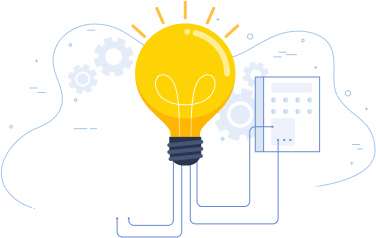
Conclusion
Real-time translation is a game-changer in the global marketplace, enabling businesses to reach a wider audience and communicate more effectively. OpenAI's language models and APIs provide powerful tools to implement real-time translation in your applications, as demonstrated in this article.
By leveraging these tools and customizing the code to your needs, you can break down language barriers and expand your business's reach in the global marketplace.