7 Easy Ways to Run an LLM Locally
Running large language models (LLMs) locally offers advantages like control, privacy, and customization. This article explores seven methods, including using Hugging Face Transformers, Docker containers, local hardware with TensorFlow or PyTorch, FastAPI, Jupyter Notebooks, GPUs, and edge devices.
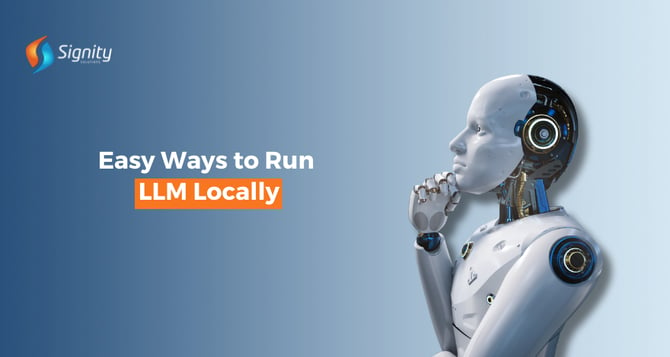
With the advancements in artificial intelligence and natural language processing, Large Language Models (LLMs) have become more accessible and powerful. These models, such as GPT-4, BERT, and T5, can generate human-like text, answer questions, and even assist with coding tasks. While many of these models are available through cloud services, running them locally can offer greater control, privacy, and customization.
Here are seven easy ways to run an LLM locally:
1. Using Pre-trained Models with Hugging Face
Hugging Face Transformers is one of the most popular libraries for working with LLMs. It provides pre-trained models that you can download and run locally. Here’s how you can get started:
Install the Library:
pip install transformers |
Download and use a Pre-Trained Model:
from transformers import pipeline generator = pipeline('text-generation', model='gpt-2') result = generator("Once upon a time,") print(result) |
This method is straightforward and ideal for those who want to quickly get up and running with an LLM without worrying about training the model from scratch.
2. Using Docker Containers
Docker containers provide a convenient way to run applications in isolated environments. Many LLMs have Docker images available, making it easy to set up and run locally:
- Install Docker from the official website.
- Pull the Docker image for your chosen LLM:
docker pull huggingface/transformers-pytorch-gpu:latest |
- Run the container:
docker run -it --rm huggingface/transformers-pytorch-gpu:latest |
Using Docker ensures that you have all the necessary dependencies and configurations in place.
3. Deploying on Local Hardware with TensorFlow or PyTorch
If you have a powerful local machine, you can run LLMs using TensorFlow or PyTorch. This approach gives you more flexibility and control over the model’s performance.
Install TensorFlow or PyTorch:
pip install tensorflow # or pip install torch |
Load a Pre-Trained Model:
import tensorflow as tf from transformers import TFAutoModelForCausalLM, AutoTokenizer tokenizer = AutoTokenizer.from_pretrained("gpt2") model = TFAutoModelForCausalLM.from_pretrained("gpt2") input_ids = tokenizer.encode("Once upon a time,", return_tensors='tf') output = model.generate(input_ids) print(tokenizer.decode(output[0], skip_special_tokens=True)) |
This method is ideal for users with experience in machine learning and who need to fine-tune models for specific tasks.
4. Using Local APIs with FastAPI
FastAPI is a modern web framework for building APIs with Python. You can create a local API to interact with your LLM:
Install FastAPI and Uvicorn:
pip install fastapi uvicorn transformers |
Create an API Endpoint:
from fastapi import FastAPI from transformers import pipeline app = FastAPI() generator = pipeline('text-generation', model='gpt-2') @app.get("/generate") def generate_text(prompt: str): return generator(prompt) if __name__ == "__main__": import uvicorn uvicorn.run(app, host="127.0.0.1", port=8000) |
Run the API Server:
uvicorn main:app --reload |
This setup allows you to send HTTP requests to your local server and get responses from the LLM.
5. Using Jupyter Notebooks
Jupyter Notebooks are great for experimenting with LLMs locally. They provide an interactive environment where you can write and execute code in cells:
Install Jupyter Notebook
pip install notebook |
Launch Jupyter Notebook
jupyter notebook |
Run your LLM Code in a Notebook Cell
from transformers import pipeline generator = pipeline('text-generation', model='gpt-2') result = generator("Once upon a time,") print(result) |
Jupyter Notebooks are particularly useful for data scientists and researchers who need to document their work.
6. Using Local GPU for Enhanced Performance
If you have a GPU on your local machine, you can leverage it to accelerate the performance of LLMs:
Install the Necessary Libraries
pip install torch torchvision torchaudio |
Ensure CUDA is installed and properly configured on your machine.
Run the LLM on the GPU
import torch from transformers import GPT2LMHeadModel, GPT2Tokenizer device = torch.device("cuda" if torch.cuda.is_available() else "cpu") model = GPT2LMHeadModel.from_pretrained("gpt2").to(device) tokenizer = GPT2Tokenizer.from_pretrained("gpt2") inputs = tokenizer("Once upon a time,", return_tensors="pt").to(device) outputs = model.generate(inputs["input_ids"]) print(tokenizer.decode(outputs[0], skip_special_tokens=True)) |
Using a GPU can significantly speed up the processing time, making it ideal for larger models and datasets.
7. Running on Edge Devices
For applications that require LLMs on edge devices like Raspberry Pi or mobile devices, you can use optimized frameworks such as TensorFlow Lite or ONNX Runtime:
- Convert your model to TensorFlow Lite or ONNX format.
- Run the model on your edge device using the respective runtime environment.
For example, to run a model on a Raspberry Pi using TensorFlow Lite:
Install TensorFlow Lite
pip install tflite-runtime |
Run the Converted Model
import tflite_runtime.interpreter as tflite from transformers import AutoTokenizer tokenizer = AutoTokenizer.from_pretrained("gpt2") model_path = "path/to/your/model.tflite" interpreter = tflite.Interpreter(model_path=model_path) interpreter.allocate_tensors() input_details = interpreter.get_input_details() output_details = interpreter.get_output_details() input_data = tokenizer.encode("Once upon a time,", return_tensors='tf') interpreter.set_tensor(input_details[0]['index'], input_data) interpreter.invoke() output_data = interpreter.get_tensor(output_details[0]['index']) print(tokenizer.decode(output_data[0], skip_special_tokens=True)) |
Running LLMs on edge devices allows for low-latency, offline applications, making it suitable for IoT and mobile applications.
How Signity Solutions Can Help
At Signity Solutions, we specialize in AI and LLM development. Our team of experts can assist you in leveraging these advanced technologies for your specific needs. Whether you need to develop custom models, optimize existing ones, or deploy them on local hardware, Signity Solutions provides end-to-end services to ensure your AI projects succeed.
Conclusion
Running LLMs locally offers numerous advantages, including greater control, privacy, and customization. By following the methods outlined in this article, you can harness the power of advanced language models right on your local machine.
Whether you are a developer looking to integrate AI into your projects, a researcher aiming to experiment with the latest models, or a business seeking customized AI solutions, running LLMs locally provides the flexibility and capabilities you need. And with the support of Signity Solutions, you can ensure your AI initiatives are both innovative and successful.